This is a docker-compose.yml file designed to allow users to run MariaDB + PHP + Apache to use for learning purposes. Below is the docker-compose file.
# This file deploys MariaDB, PMA and Apache.
# deploy this using Docker and wait a bit for it to start up.
# -----------------------------------------------------------------------------------------------
# PHP MY ADMIN: http://localhost:8090/
# TERMINAL FOR DATABASE: https://localhost:8091/ user is kasm_user and password is 'password'.
# FILE UPLOAD TO APACHE: http://localhost:8094/ user is admin and password is admin
# APACHE: http://localhost:8093/
# -----------------------------------------------------------------------------------------------
# User is ROOT and password is notSecureChangeMe and database is db
# Note: you dont have to change the password unless this will be exposed to the internet.
services:
db:
container_name: database
image: mariadb:10.11
restart: always
volumes:
- mysql_lessons:/var/lib/mysql
environment:
MYSQL_ROOT_PASSWORD: notSecureChangeMe
post_start:
- command: ["/bin/bash", "-c", "apt update && apt install openssh-server -y && echo 'root:root' | chpasswd && echo 'Changed root password' && echo PermitRootLogin yes >> /etc/ssh/sshd_config && service ssh restart && echo mysql -u root -pnotSecureChangeMe >> /root/.bashrc"]
phpmyadmin:
container_name: phpmyadmin
image: phpmyadmin
restart: always
ports:
- 8090:80
depends_on:
- db
environment:
- PMA_ARBITRARY=1
- PMA_HOST=db
- MYSQL_ROOT_PASSWORD=notSecureChangeMe
- PMA_USER=root
- PMA_PASSWORD=notSecureChangeMe
links:
- db:db
terminal-db:
image: kasmweb/terminal:1.16.0
container_name: mysql-term
restart: always
depends_on:
- db
ports:
- 8091:6901
environment:
- VNC_PW=password
- SHELL_EXEC=sshpass -p 'root' ssh -o StrictHostKeyChecking=no root@db
apache:
container_name: webserver
image: 'php:7.4-apache'
restart: always
ports:
- '8093:80'
volumes:
- apache:/var/www/html
links:
- db:db
command: ["/bin/bash", "-c", "docker-php-ext-install mysqli && exec apache2-foreground"]
uploader:
image: filebrowser/filebrowser:latest
container_name: uploader
ports:
- "8094:80" # Expose the web interface on port 8080
volumes:
- apache:/srv
post_start:
- command: ["/bin/bash", "-c", "chmod 777 /srv/*"]
environment:
- FB_NO_AUTH=true
restart: unless-stopped
volumes:
mysql_lessons:
apache:
Save this on your desktop as docker-compose-lamp.yml.
Recommended Installation: Use Local Docker
If you have Docker installed on your PC: shift + right clicking
and selecting “Open PowerShell Window here”:
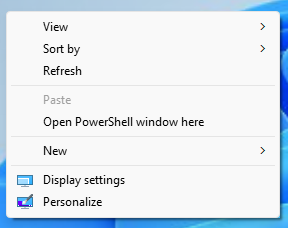
Type to run docker containers: docker compose -f docker-compose-lamp.yml up -d
Type to stop docker containers: docker compose -f docker-compose-lamp.yml
It may take a while before they start, so be patient. If it’s done, it will look something like this:
(other docker messages)[+] Running 6/6
✔ Network desktop_default Created 0.1s
✔ Container uploader Started 0.9s
✔ Container database Started 28.3s
✔ Container mysql-term Started 29.0s
✔ Container webserver Started 28.7s
✔ Container phpmyadmin Started 29.0s
PS C:\Users\Desktop>
Now you can use the localhost urls to access Docker. Jump to bottom.
On the cloud, use Google Cloud or Play with Docker.
Option 1: Play with Docker (not recommended)
Note that cloud options may have limited working hours and may not be available at all times. For Play with docker:
Click Login:
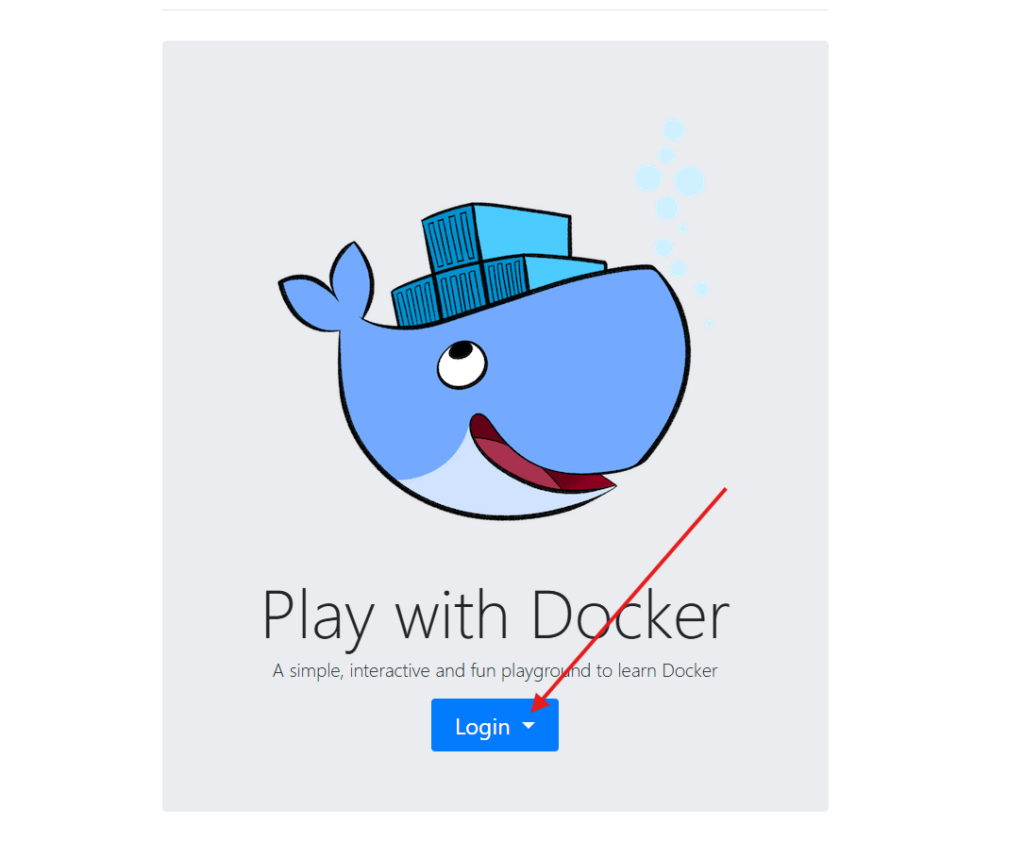
Click Docker:
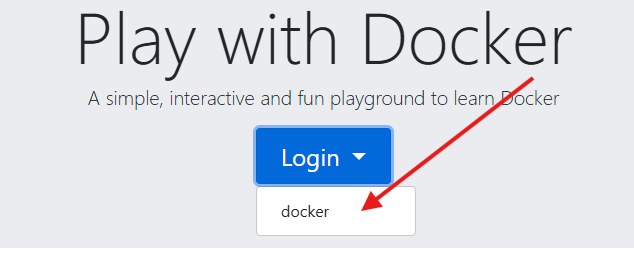
A window will popup to allow you to sign in with Docker.
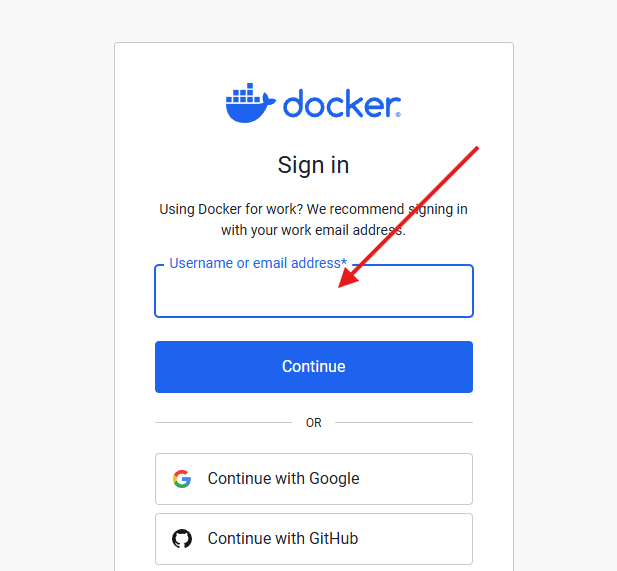
You will be redirected to Docker. Close the popup and select Docker on the login page again.
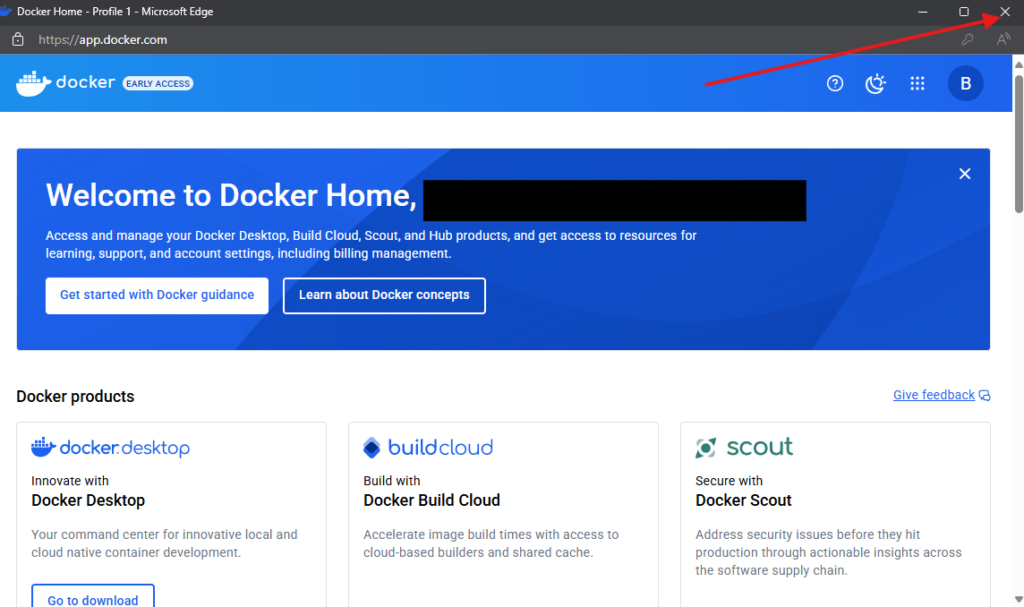
Click Login:
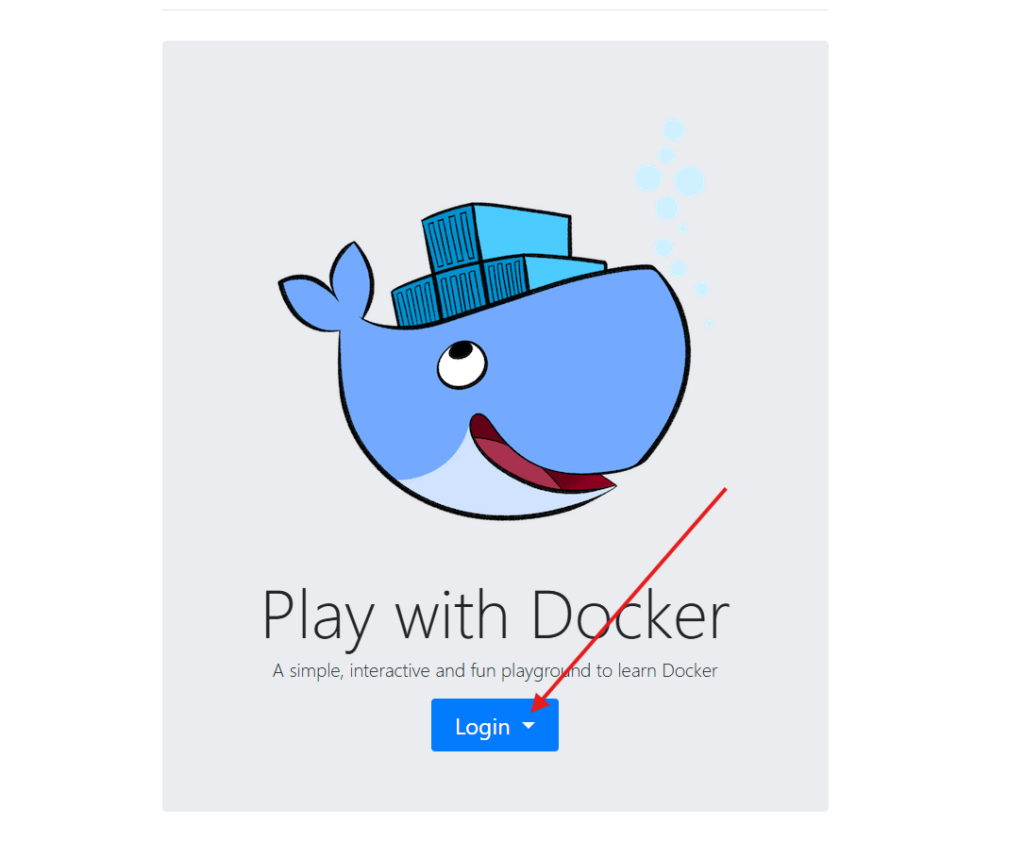
Click Docker:
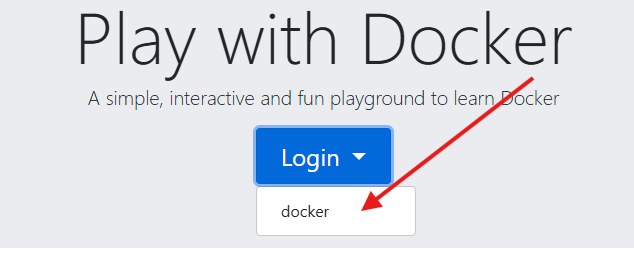
You will then see this.
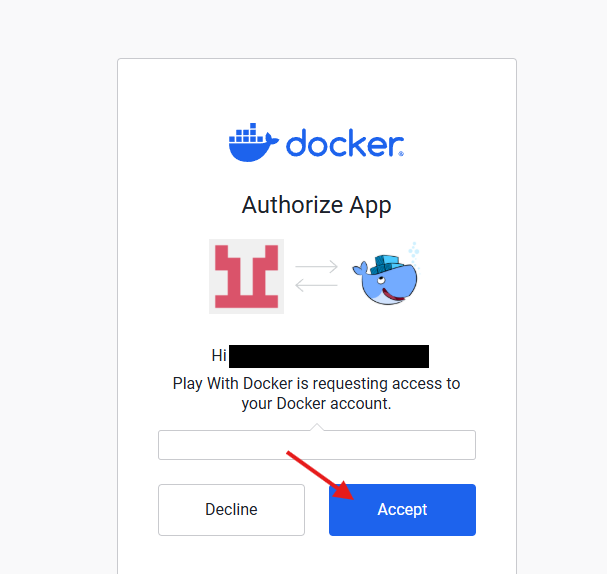
Click Accept.
Now click Start:
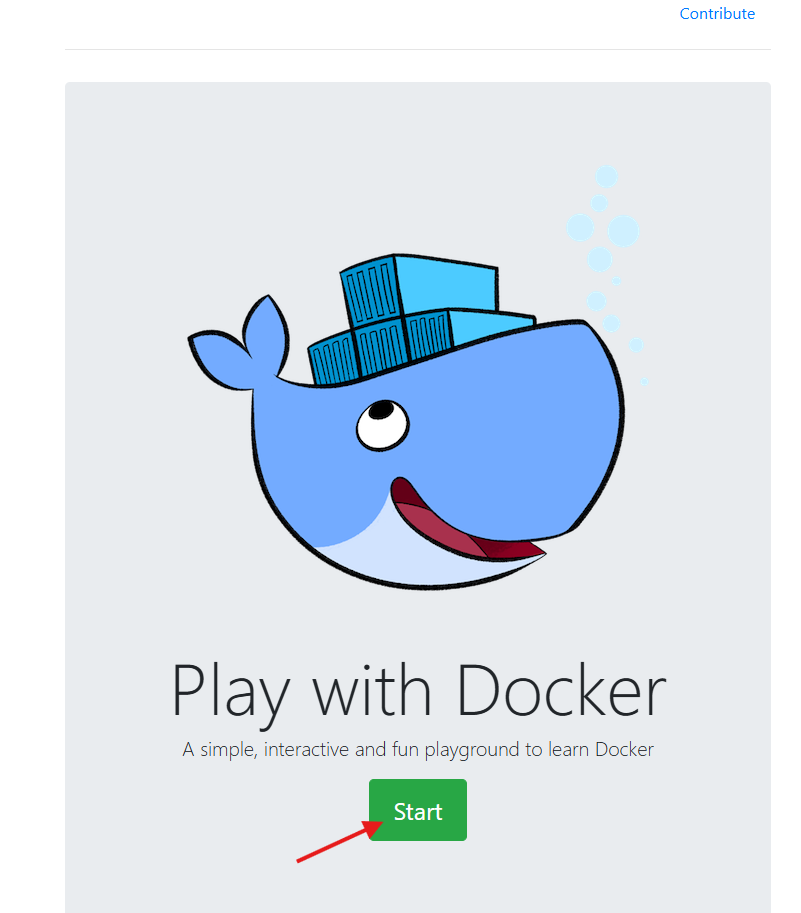
You will be redirected here:
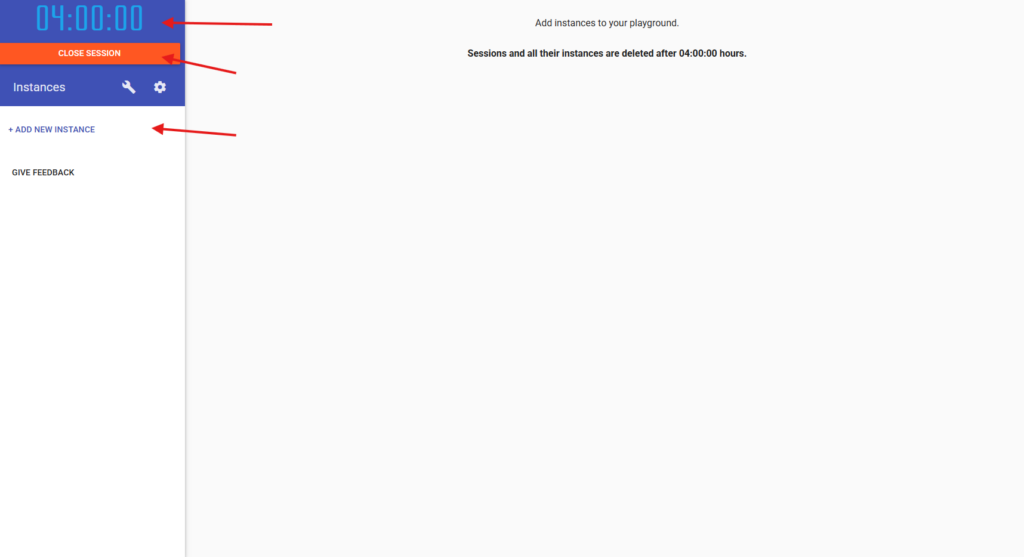
You can see session time and your instances.
Click New Instance.
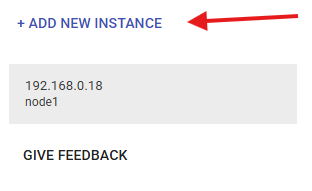
You will then see node1.
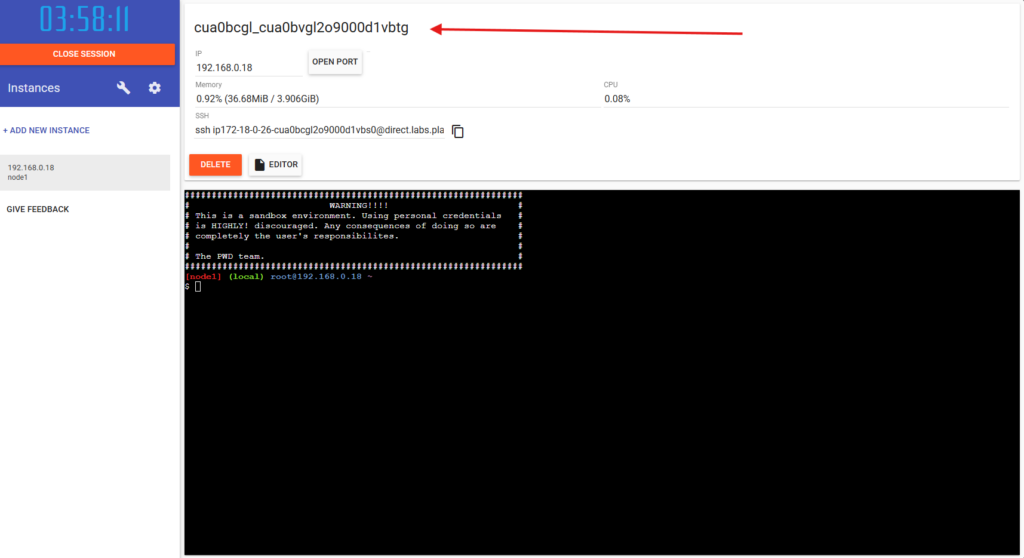
Click the terminal (the black box with text) and type in: “touch docker-compose.yml
“
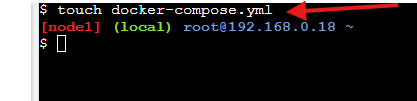
Click the editor button:

A popup window will appear. Click docker-compose.yml
.
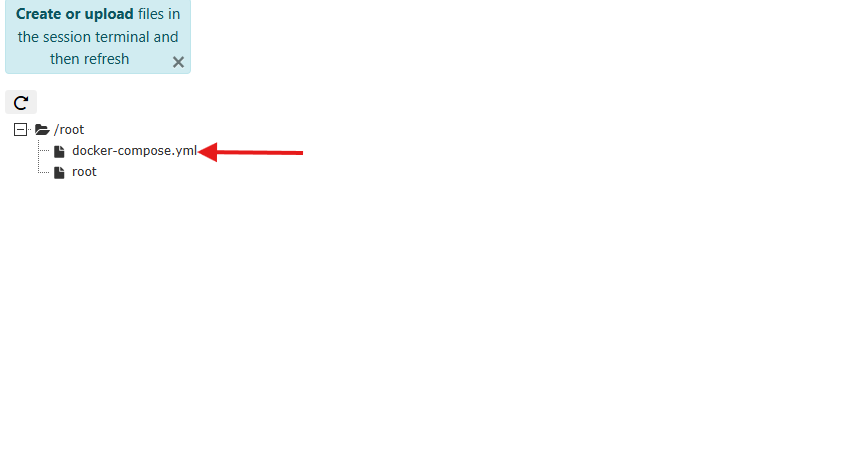
Paste my docker file into this file and click Save.
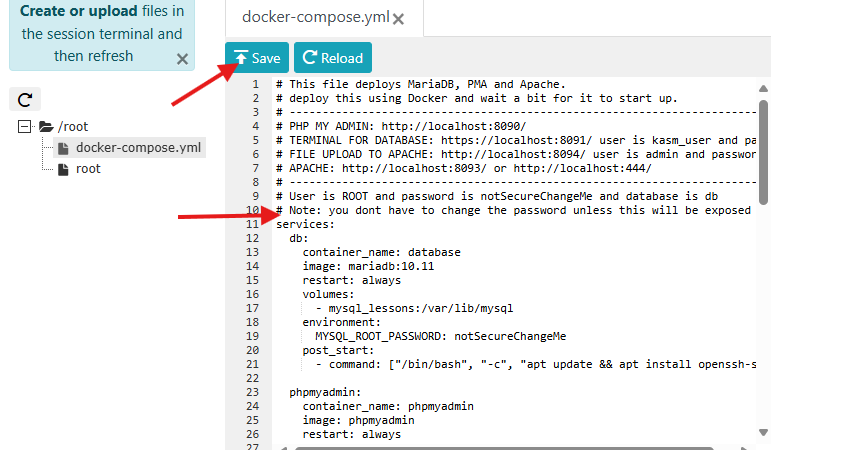
Close the popup and in the terminal type in docker-compose up -
d.
If you did it right you should see it loading like this:
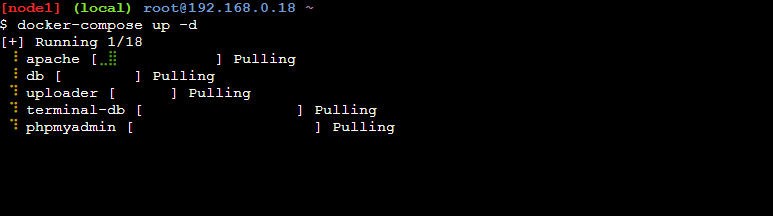
Be patient while the docker containers download.
Even if it seems stuck, its actively processing data.
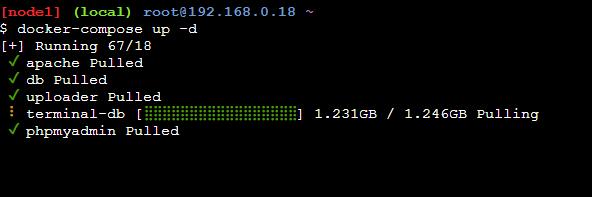
Try to visit your instance by clicking Open Port and typing in 8090.
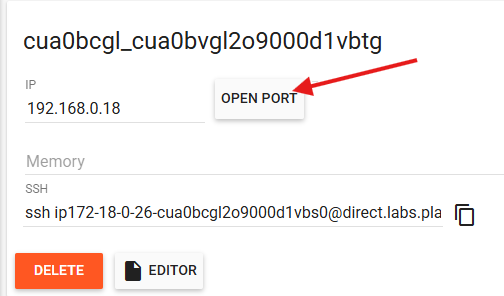
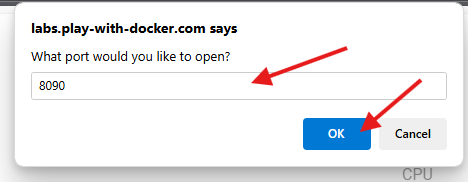
8090 is PhpMyAdmin. By opening it you should see a page simlar to this:
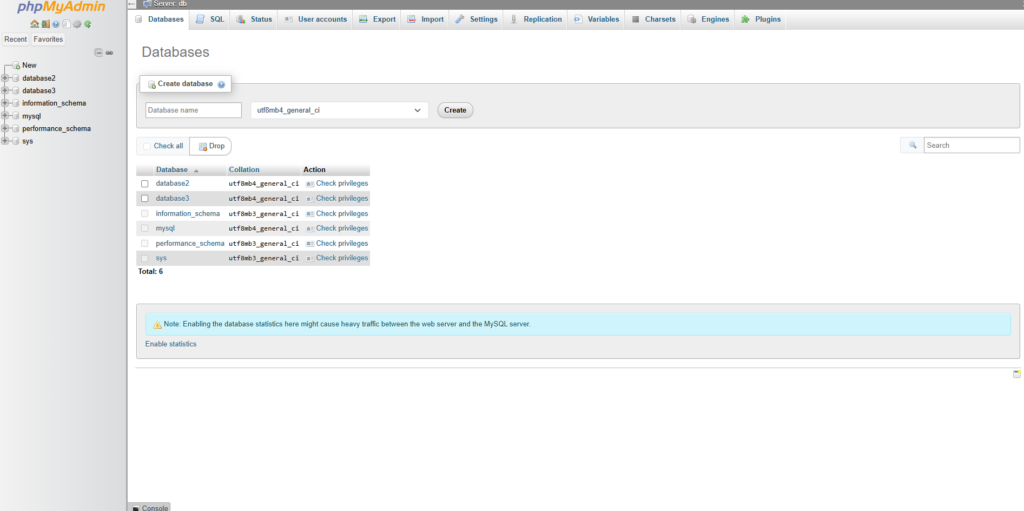
Option 2: Google Cloud:
As for Google Cloud:
Go to my link: Cloud Computing Services | Google Cloud
Click on Console on top left:
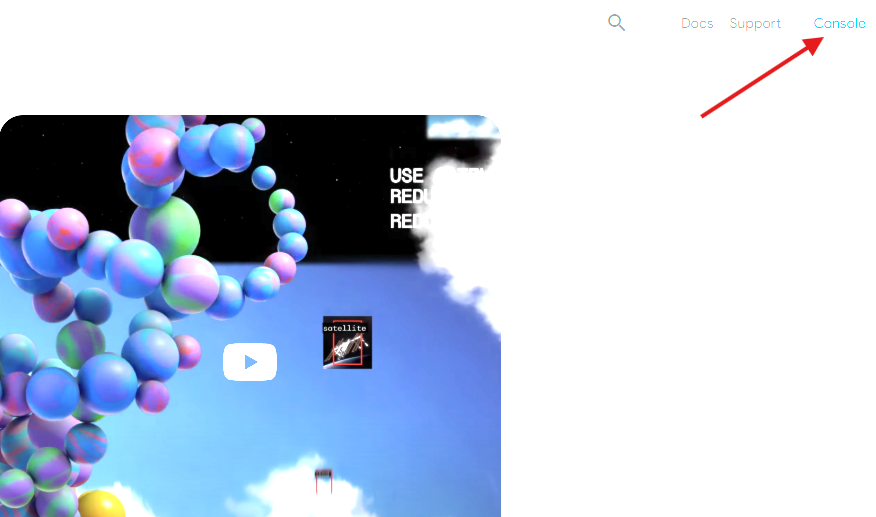
Now click on the honeycomb looking selector:
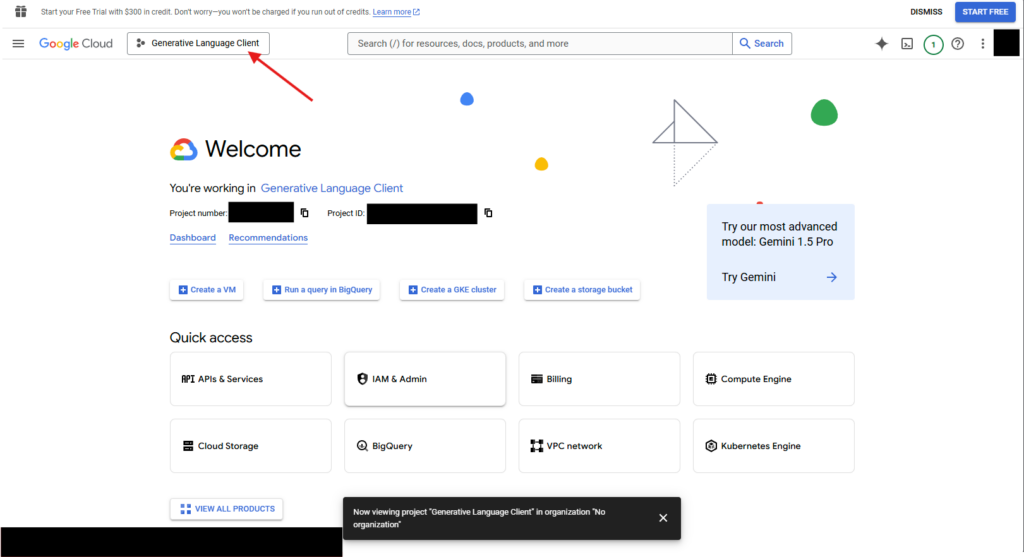
Click on new project:
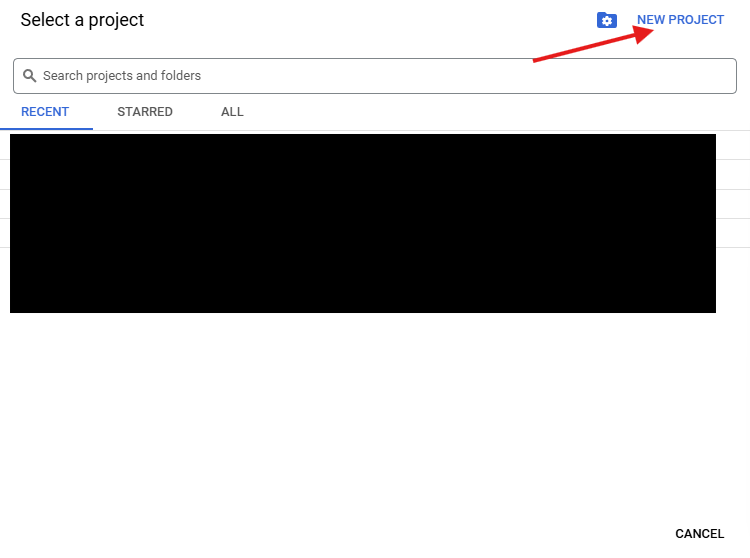
Call it something like Code space:
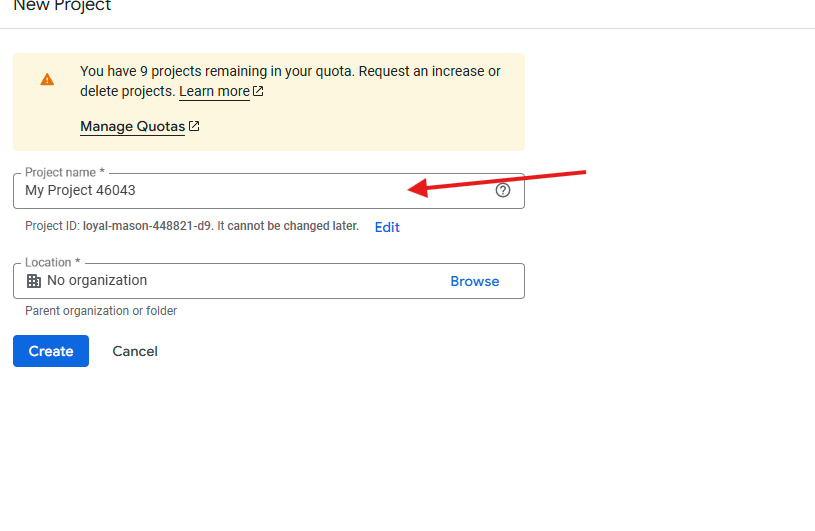
And click Create.
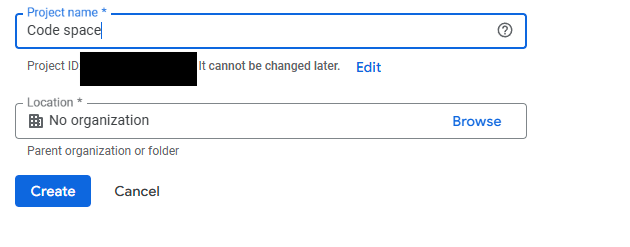
Once you are in your project, click on the Cloud Shell button:
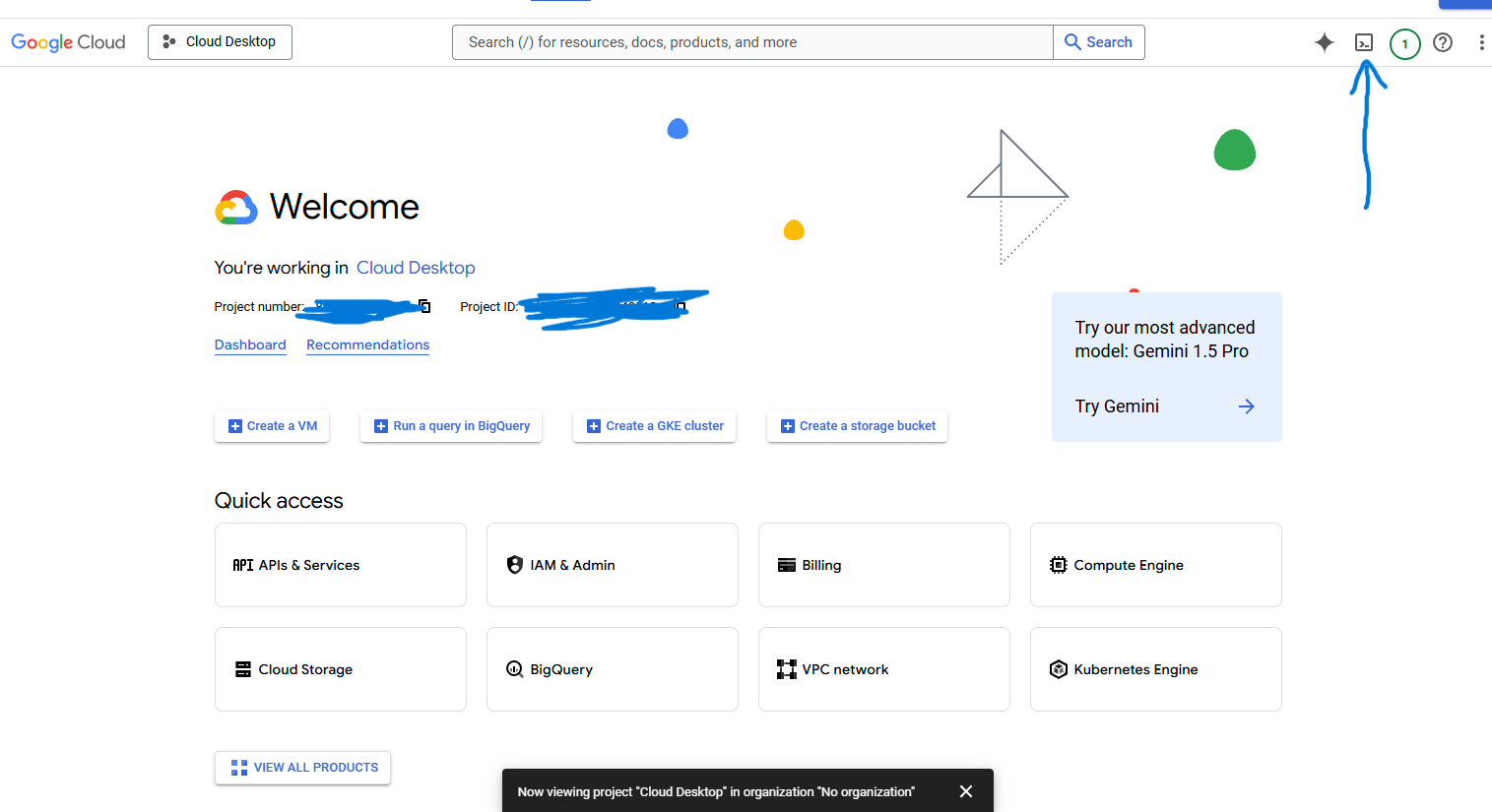
From here, click on the three dots:
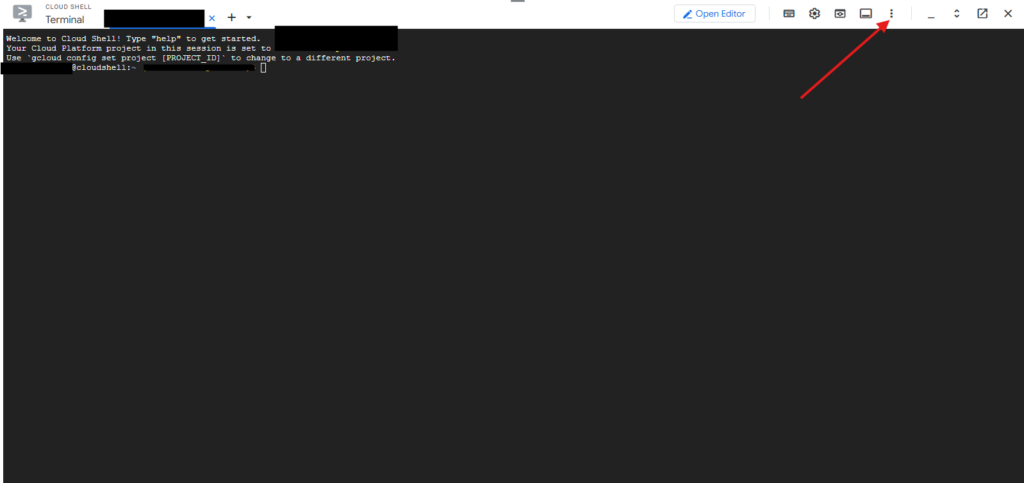
Click on Upload:
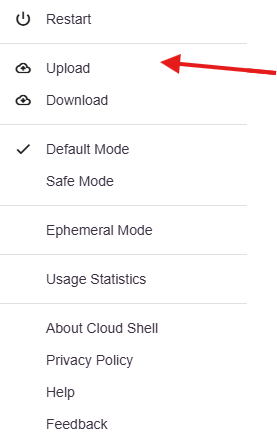
Here, click Choose Files:
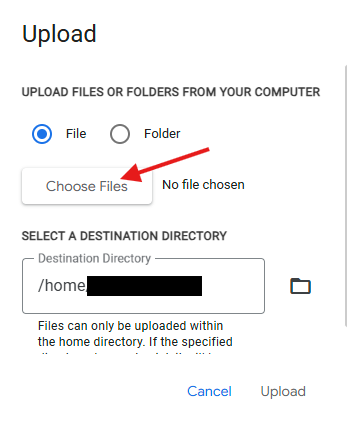
Now upload the file that you saved on your computer from the beginning.
Now click Upload.
This is similar to the PC method. Now type in docker compose -f docker-compose-lamp.yml up -d
and hit Enter.
You should see this:
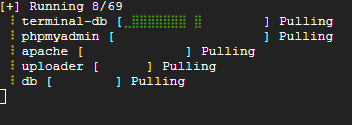
Slowly, your containers will download and you will be able to access them once we set up forwarding.
When its done you will be returned back to the command line:
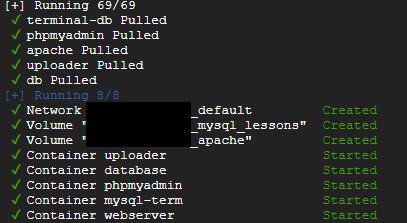
Now click this icon on the top left:
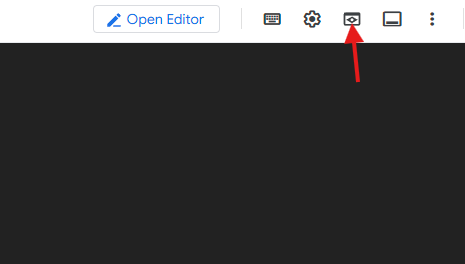
Now you click this:
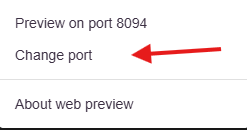
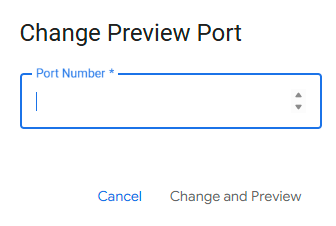
And you choose a port from the available:
# PHP MY ADMIN: http://localhost:8090/
# TERMINAL FOR DATABASE: https://localhost:8091/ user is kasm_user and password is 'password'.
# FILE UPLOAD TO APACHE: http://localhost:8094/ user is admin and password is admin
# APACHE: http://localhost:8093/
And type it in and click change and preview, and keep doing this until you open up all the ports as new tabs.
Once you are ready to shut down, type in: docker compose -f docker-compose-lamp.yml down
And close out the tab.
How to use:
Let’s first review phpMyAdmin:
Opening it in your desired workspace, you see this page:
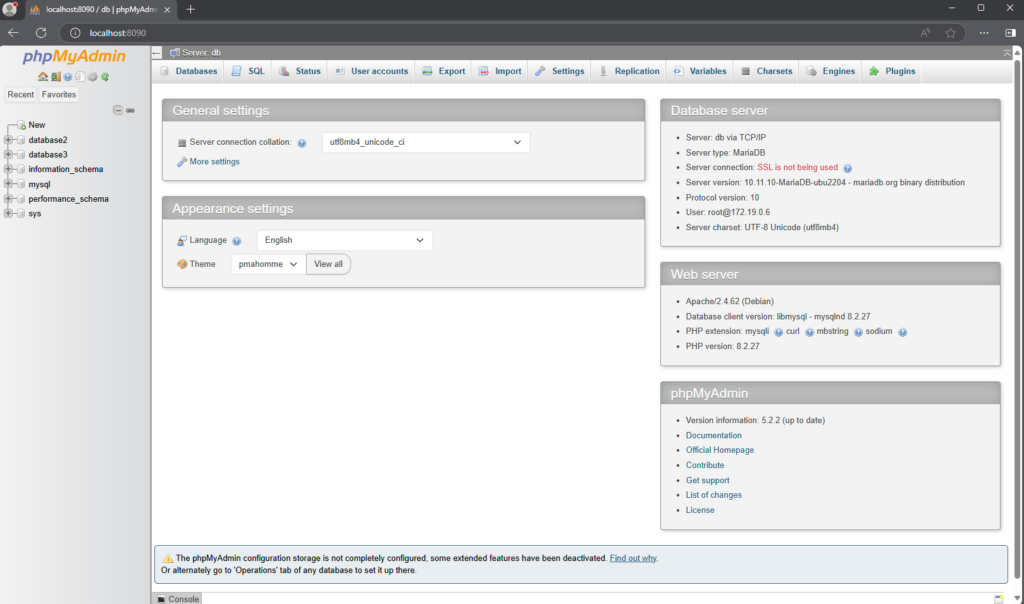
From here you can use Phpmyadmin as normal.
Opening the terminal for MariaDB on https://localhost:8091 shows us this:
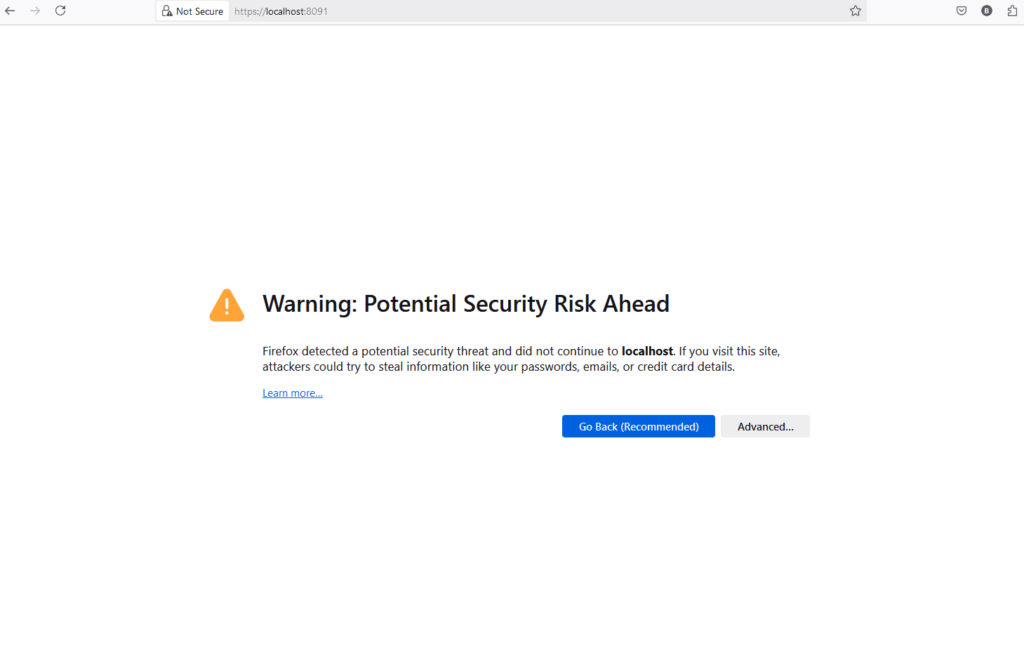
This is because the webpage uses self signed certificates. Simply click on Advanced and then “Accept the risk and Continue”
You will be them prompted to sign in:
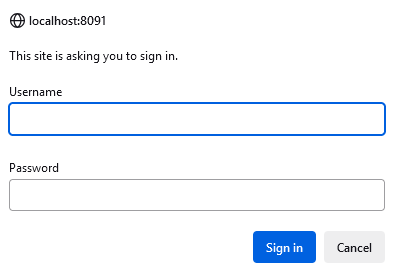
Username: kasm_user
Password: password
You will then be greeted by the MariaDB command line:
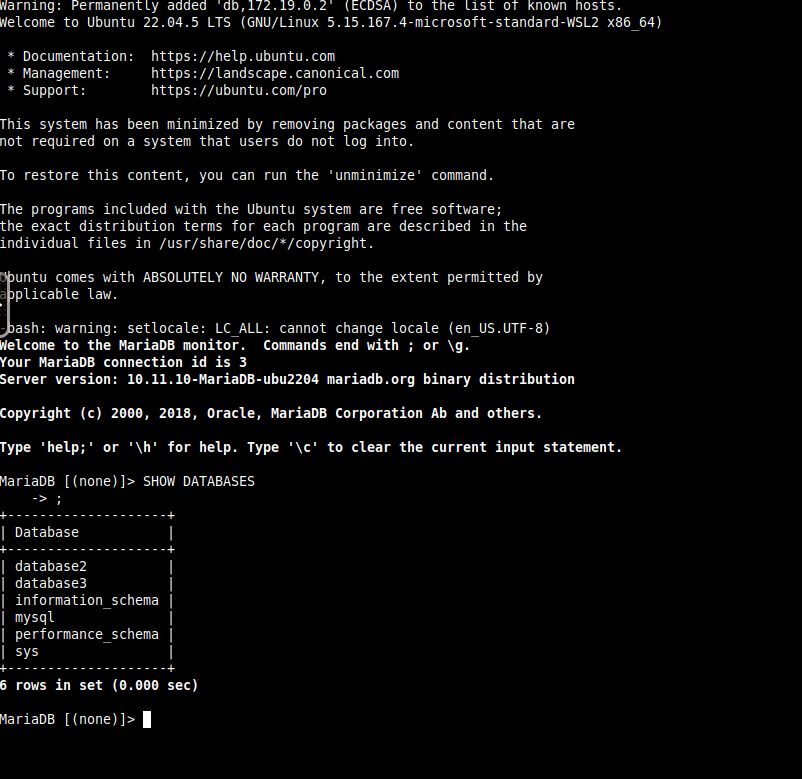
From here you can execute SQL Commands.
Opening http://localhost:8093 shows us the Apache Server. If you have nothing loaded, it will show “It works!” message.
I recommend you use this starter code to check if we are able to use PHP and MariaDB:
<?php
print "Hello From PHP \n!";
$servername = "db";
$username = "root";
$password = "notSecureChangeMe";
// Create connection
$conn = new mysqli($servername, $username, $password);
// Check connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
echo "Connected successfully to the database server.<br>";
// List databases
$result = $conn->query("SHOW DATABASES");
if ($result->num_rows > 0) {
echo "Databases:<br>";
while ($row = $result->fetch_assoc()) {
echo "- " . $row['Database'] . "<br>";
}
} else {
echo "No databases found.";
}
$conn->close();
?>
Hello from HTML!
If you get as output this:
Hello From PHP !Connected successfully to the database server.
Databases:
– information_schema
– mysql
– performance_schema
– sys
Hello from HTML!
or something slightly different, it means PHP and SQL is working.
Now, to upload files we open: http://localhost:8094/
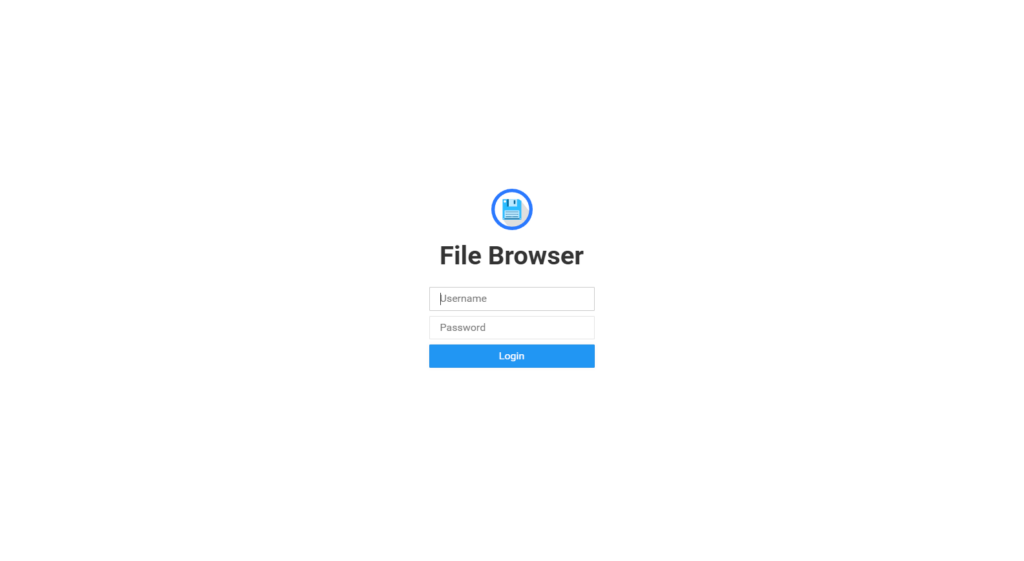
Username: admin
Password: admin
You can use this utility to upload files to Apache.
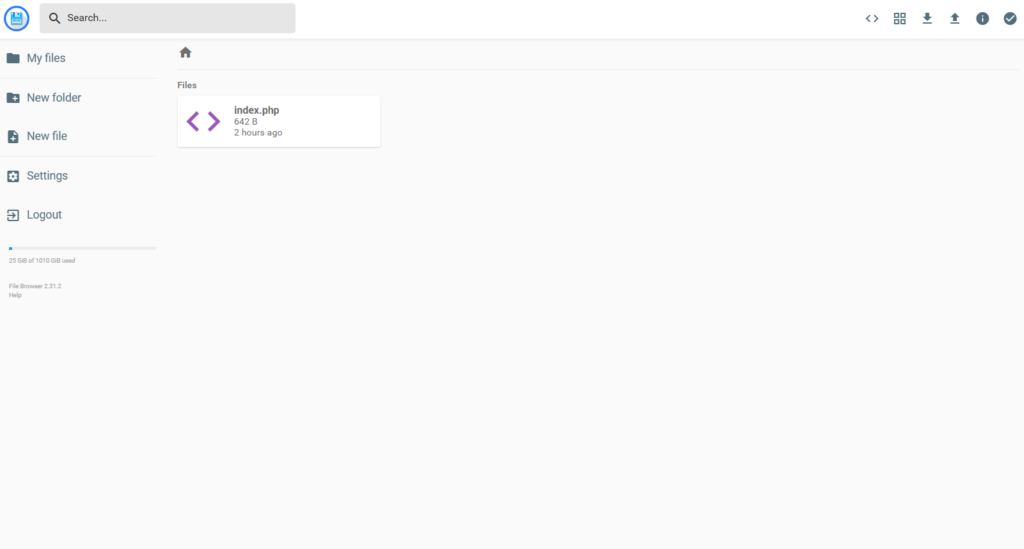
Note that this setup is not production ready – and so it is not recommended to be exposed to the web.